This is a post for an encryption program written in Python3 as a project to showcase and grow my knowledge in python, encryption, encoding, and Linux/Windows command line interface (CLI) implementations to code.
This project will be ongoing and each section will have a date marking the date of the posting. The actual post date will represent the creation of the post, not the most recent entry.
The files can be accessed from Github and the title of each update will link directly to that version of the program.
Humble Beginnings: XOR (June 18th 2023)
As someone with a intermediate understanding of python writing a simple program to encode messages using an XOR cipher was not to difficult, only running into common problems (spelling, capitalization, etc.).
This program started with the idea of a simple and fun way to send messages back in forth with a friend in a multi-person Discord server without others being able to understand what was being said.
def xor(msg, key = 'default', encode = False, decode = False):
if encode:
encrypted = ""
key_index = 0
for char in msg:
#convert character to ascii code
char_code = ord(char)
#get the corresponding key char
key_char = key[key_index % len(key)]
#convert key char to ascii code
key_code = ord(key_char)
#XOR with key
encrypted_char = char_code ^ key_code
#convert enc ascii back to char
encrypted += chr(encrypted_char)
key_index += 1
return encrypted
if decode:
decrypted = ""
key_index = 0
for char in msg:
#convert char to ascii
char_code = ord(char)
#get the corresponding key char
key_char = key[key_index % len(key)]
#convert key char to ascii code
key_code = ord(key_char)
#XOR with key
decrypted_char = char_code ^ key_code
#convert dec ascii back to char
decrypted += chr(decrypted_char)
key_index += 1
return decrypted
super_secret = "This is a secret message"
encrypted_data = xor(super_secret, "password", encode = True)
decrypted_data = xor(encrypted_data, "password", decode = True)
print("the cipher text is: ")
print(encrypted_data)
print("The plain text is: ")
print(decrypted_data)
Problems
There were few problems that were run into during this section of the program, a few being:
- The first issue was a TypeError where the original code didn’t have the section of key iteration and casting it to its ASCII value. This error was thrown because it was expecting a integer but was being supplied a string
- The second part of this error was when I changed the key to its ASCII value it was giving the error when using the ord function as I was supplying the entire key instead of iterating letter by letter.
Lessons Learned
I am a firm believer in the John C. Maxwell quote “You never really know something until you [could] teach it to someone else.” So I try to make sure that I understand why and how things work before considering a problem or project finished.
- I learned in more depth how the XOR and other logic gate functions work
- I continued to grow my python knowledge
Next Steps
The next steps I hope to add the ability to add the message, key, and encode/decode values from the CLI and adding another type of encryption or encoding.
This code also only reliably works in a sandbox where it feeds it straight from encode to decode through the variables, so the next version will encode the data to base64 so that it can be transmitted.
Better Experience (June 19, 2023)
This version of the program added Base64 encoding to the program so that the encrypted text was able to be copied and pasted and sent in common text.
A simple input based menu was also created for the program so the values didn’t need to be hard coded.
The files were also added to Github so that the updates can be seen and older versions of the program can be viewed.
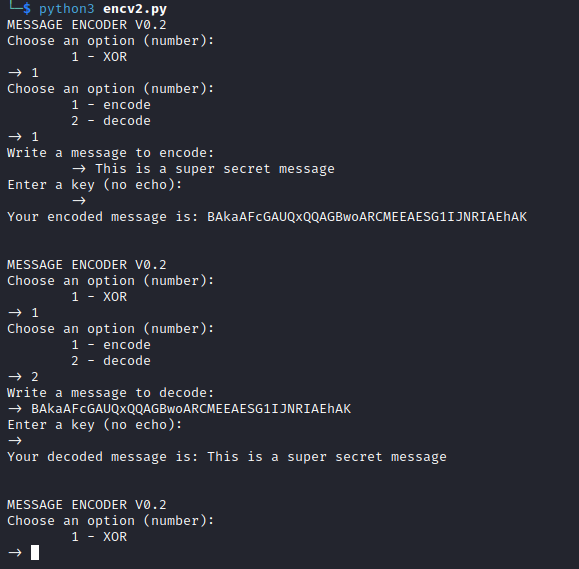
Problems
I only ran in to minor problems in this version of the program, all of which can be chalked up to me stretching my programming legs.
Lessons Learned
- I brushed up on my input based menu skills
- I learned about the getpass library and implemented it for the key
- I read up on and added the base64 library
Next Steps
I plan to add another, more complicated to implement form of encryption.